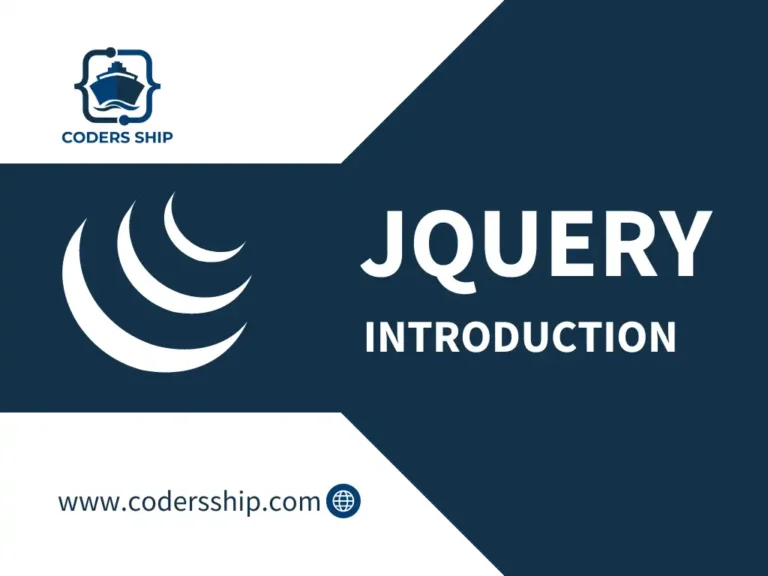
Introduction to jQuery
This is where jQuery steps in as a powerful tool to simplify JavaScript development. In this article, we’ll explore what jQuery is, its advantages, and how it simplifies JavaScript development.
What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies things like HTML document traversal and manipulation, event handling, animation, and AJAX interaction for rapid web development. Essentially, jQuery allows developers to write less code while achieving more.
At its core, jQuery revolves around the concept of selecting HTML elements and performing actions on them using a concise syntax. It provides a set of methods that make common tasks, such as DOM manipulation and event handling, more straightforward and efficient.
Advantages of Using jQuery
Simplified DOM Manipulation:
One of the most significant advantages of jQuery is its ability to manipulate the Document Object Model (DOM) effortlessly. With jQuery, you can easily select DOM elements using CSS-like selectors and perform actions like adding or removing classes, changing styles, or modifying content with minimal code.
// Example: Changing the text color of all paragraphs with class 'highlight'
$(".highlight").css("color", "red");
Cross-browser Compatibility:
jQuery abstracts away many of the inconsistencies and quirks present in different browsers, providing a consistent API that works seamlessly across various platforms. This ensures that your web applications behave predictably regardless of the browser being used.
Event Handling Simplification:
Handling events in JavaScript can be cumbersome, especially when dealing with cross-browser compatibility. jQuery simplifies event handling by providing methods like on() and trigger() that abstract away the complexities, allowing developers to focus on the application logic.
// Example: Handling a click event
$("button").on("click", function() {
console.log("Button clicked!");
});
AJAX Support:
jQuery simplifies asynchronous HTTP requests (AJAX) with its AJAX methods, such as $.ajax(), $.get(), and $.post(). These methods streamline the process of sending and receiving data from a server, making it easier to build dynamic web applications that communicate with backend services.
// Example: Fetching data from a server using AJAX
$.get("https://api.example.com/data", function(data) {
console.log("Data received:", data);
});
Animation Effects:
jQuery provides built-in methods for creating smooth and visually appealing animations on web pages. Whether it’s fading elements in and out, sliding them up and down, or creating custom animations, jQuery’s animation capabilities allow developers to enhance the user experience without diving into complex CSS or JavaScript code.
// Example: Fading out an element over 1 second
$("#myElement").fadeOut(1000);
How jQuery Simplifies JavaScript Development
jQuery simplifies JavaScript development in several ways:
Concise Syntax:
jQuery’s intuitive API and concise syntax reduce the amount of code required to achieve common tasks, resulting in cleaner and more maintainable codebases.
Abstraction of Complexities:
By abstracting away browser quirks and inconsistencies, jQuery allows developers to focus on building features rather than worrying about cross-browser compatibility issues.
Extensibility:
jQuery is highly extensible, with a vast ecosystem of plugins and extensions available to enhance its functionality. This enables developers to leverage existing solutions and build upon them to meet their specific requirements.
Rapid Prototyping:
With its ease of use and extensive feature set, jQuery is an excellent choice for rapid prototyping and proof-of-concept development. Developers can quickly iterate on ideas and experiment with different UI interactions without getting bogged down by low-level details.
Community Support:
jQuery has a large and active community of developers who contribute plugins, tutorials, and support resources. This vibrant ecosystem makes it easy for developers to find solutions to their problems and stay updated with the latest best practices.
Conclusion
jQuery remains a valuable tool in the toolkit of web developers, even as newer frameworks and libraries emerge. Its simplicity, versatility, and widespread adoption make it an excellent choice for building dynamic and interactive web applications. By leveraging jQuery’s power, developers can streamline JavaScript development and deliver rich user experiences with less effort. Whether you’re a seasoned developer or just starting out, jQuery is worth exploring for its ability to simplify and enhance web development workflows.